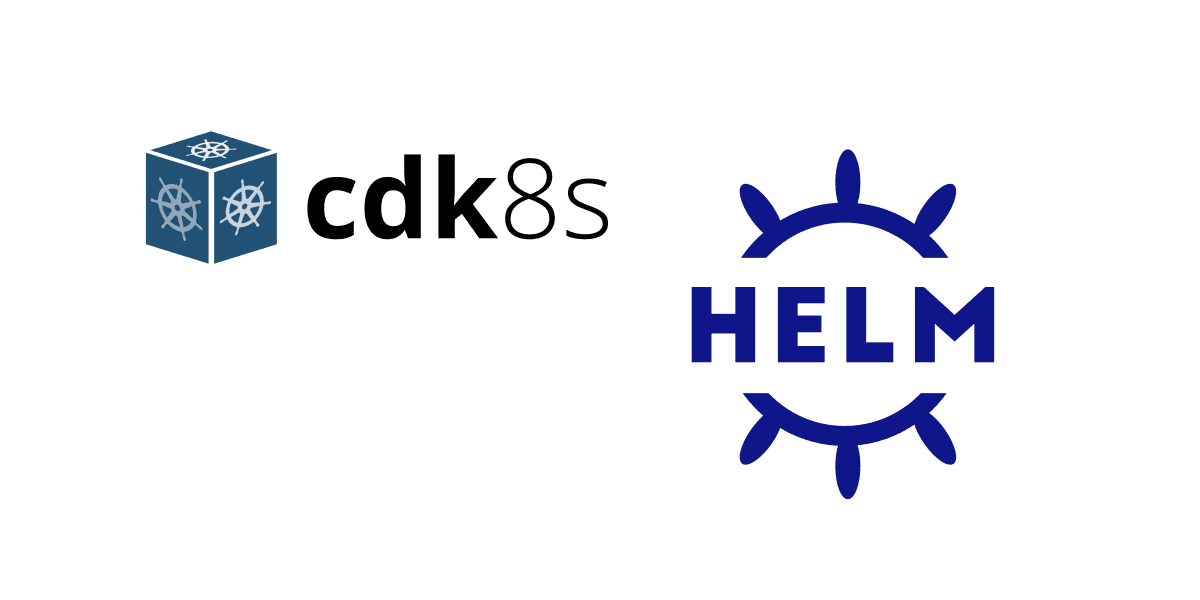
Generate a custom type-safe Helm Chart construct with cdk8s
Cloud DevOps @ smallfries.digital
Date: 2023-11-02
Cloud Development Kit for Kubernetes (cdk8s) is a software development framework for defining Kubernetes applications and reusable abstractions using familiar programming languages and rich object-oriented APIs. cdk8s generates pure Kubernetes YAML - you can use cdk8s to define applications for any Kubernetes cluster running anywhere.
Recently the cdk8s team has expanded the capabilities of the "cdk8s import" command, simplifying the process to incorporate Helm charts. Providing a Helm Chart URL to the "cdk8s import" command will auto-generate a custom type-safe construct for you that represents the Helm Chart in question.
Let's start by initializing a new cdk8s project in an empty directory:
mkdir cdk8simporthelm && cd cdk8simporthelm
cdk8s init typescript-app
You should see the following basic structure of a cdk8s application with the constructs and cdk8s libraries:
import { Construct } from 'constructs';
import { App, Chart, ChartProps } from 'cdk8s';
export class MyChart extends Chart {
constructor(scope: Construct, id: string, props: ChartProps = { }) {
super(scope, id, props);
// define resources here
}
}
const app = new App();
new MyChart(app, 'cdk8simporthelm');
app.synth();
});
Now let's go ahead and import the Bitnami Redis chart:
cdk8s import helm:https://charts.bitnami.com/bitnami/redis@18.2.0
Moving back to our main.ts file we can now import the new Redis module that was generated in the previous step:
import { Construct } from "constructs";
import { App, Chart, ChartProps } from "cdk8s";
import { Redis, RedisArchitecture } from "./imports/redis";
export class MyChart extends Chart {
constructor(scope: Construct, id: string, props: ChartProps = {}) {
super(scope, id, props);
new Redis(this, "Redis", {
values: {
architecture: RedisArchitecture.REPLICATION, // <------- type safe property
},
});
}
}
const app = new App();
new MyChart(app, "cdk8simporthelm");
app.synth();
After we're done adding anything else we need in addition to what's above, let's synthesize the k8s YAML manifest:
cdk8s synth
You should now have your manifest generated in the dist subfolder and you can run kubectl apply as you would with any other manifest.
You can find the source code repo for this blog post here: https://github.com/smallfriesdigital/cdk8s-helm-import
To access more info about Values Schema, Additional Values, Globals and Dependencies visit https://cdk8s.io/docs/latest/cli/import/#helm-charts